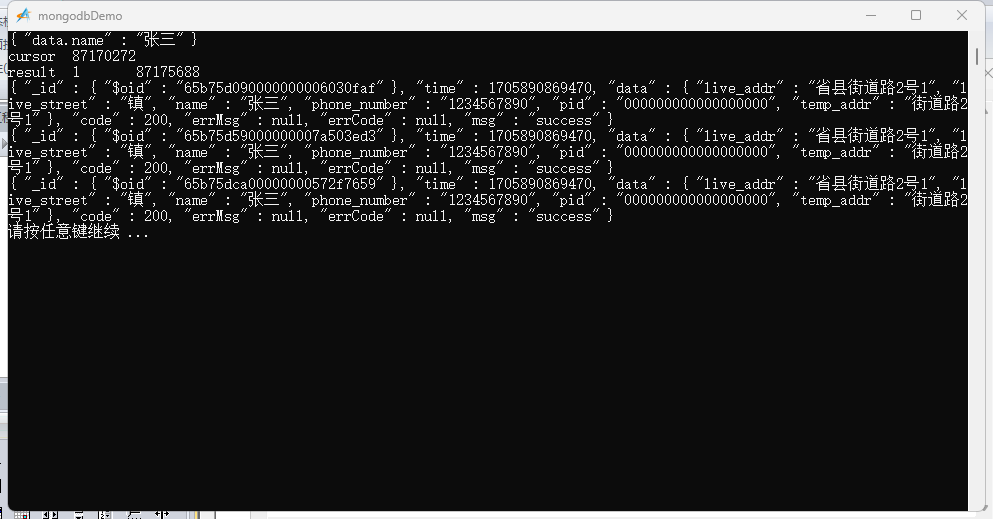
import win.ui;
/*DSG{{*/
var winform = win.form(text="mongodbDemo";right=759;bottom=469)
winform.add(
edit={cls="edit";left=15;top=11;right=743;bottom=446;edge=1;hscroll=1;multiline=1;vscroll=1;z=1}
)
/*}}*/
winform.show();
import process.popen
import fsys
var path=io.fullpath("/mongod.exe")
fsys.createDir("c:\testdata")
var prcs = process.popen(path,"--dbpath c:\testdata")
prcs.logResponse(winform.edit);
sleep(3000)
import console;
dll := raw.loadDll("\libmongoc-1.0.dll", ,"cdecl");
mongoc_init = dll.api("mongoc_init", "bool()");
mongoc_cleanup = dll.api("mongoc_cleanup", "bool()");
mongoc_client_new = dll.api("mongoc_client_new", "int(string a1)");
mongoc_client_destroy = dll.api("mongoc_client_destroy", "int(int a1)");
mongoc_client_get_database = dll.api("mongoc_client_get_database", "int(int a1,string a2)");
mongoc_database_destroy = dll.api("mongoc_database_destroy", "int(int a1)");
mongoc_client_get_collection = dll.api("mongoc_client_get_collection", "int(int a1,string a2,string a3)");
mongoc_collection_destroy = dll.api("mongoc_collection_destroy", "int(int a1)");
mongoc_collection_find = dll.api("mongoc_collection_find", "int(int a1,int a2,int a3,int a4,int a5,int a6,int a7,int a8)");
mongoc_cursor_next = dll.api("mongoc_cursor_next", "byte(int a1,int &a2)");
mongoc_collection_insert = dll.api("mongoc_collection_insert", "byte(int a1,int a2,int a3,int a4,struct &a5)");
mongoc_collection_update =dll.api("mongoc_collection_update", "byte(int a1,int a2,int a3,int a4,int a5,struct &a6)");
mongoc_collection_remove = dll.api("mongoc_collection_remove", "byte(int a1,int a2,int a3,int a4,struct &a5)");
mongoc_client_command_simple = dll.api("mongoc_client_command_simple", "byte(int a1,string a2,int a3,int a4,int a5,struct &a6)");
mongoc_collection_command_simple = dll.api("mongoc_collection_command_simple", "byte(int a1,int a2,int a3,int a4,struct &a5)");
mongoc_database_command_simple = dll.api("mongoc_database_command_simple", "int(int a1,int a2,int a3,int a4,int a5,struct &a6)");
bdll := raw.loadDll("\libbson-1.0.dll",,"cdecl");
bcon_new = bdll.api("bcon_new", "int()");
bson_destroy = bdll.api("bson_destroy", "int(int a1)");
bson_oid_init = bdll.api("bson_oid_init", "int(int &a1,int a2)");
bson_as_json = bdll.api("bson_as_json", "string(int a1,int a2)");
bson_append_utf8 =bdll.api("bson_append_utf8", "int(int a1,string a2,int a3,string a4,int a5)");
bson_append_oid =bdll.api("bson_append_oid", "int(int a1,string a2,int a3,int &a4)");
bson_new_from_json =bdll.api("bson_new_from_json", "int(string a1,int a2,struct &a3)");
class Bsonerr{
INT domain=0;
INT code=0;
byte err[504];
}
bserr=Bsonerr();
//初始化
if (mongoc_init()) console.log("初始化成功");
//连接mongodb获得client句柄
client = mongoc_client_new("mongodb://localhost:27017");
console.log("client", client);
//执行client命令操作(ping)
import web.json
var tab={ping=1}
json=web.json.stringify(tab);
commad=bson_new_from_json(json,-1,bserr);
if(!commad){
console.log(commad,"bson",bserr.code,bserr.err)
}
var a=bcon_new();
r=mongoc_client_command_simple (client, "admin", commad, 0, a, bserr);
bson_destroy(commad)
console.logPause(bson_as_json(a,0));
if(!r){
console.log(r,bserr.code,bserr.err);
console.logPause("连接错误,程序将关闭");
//销毁client句柄
mongoc_client_destroy(client);
//销毁初始化
ab = mongoc_cleanup();
return win.quitMessage();
}
//连接数据库获得database句柄
database = mongoc_client_get_database(client, "test");
console.log("database", database);
//连接集合获得collection句柄
collection = mongoc_client_get_collection(client, "testa", "demo");
console.log("collection", collection);
console.pause();
//创建insert空bson
insert=bcon_new();
var a=0;
//获取oid
r,a=bson_oid_init(a,0);
//空bson追加_id字段和oid值
bson_append_oid(insert,"_id",-1,a);
//bson_append_utf8(insert,"_id",-1,"65af647f0000000080eef4a2",-1)
//json追加aaa字段和bbbbbbb值
bson_append_utf8(insert,"aaa",-1,"bbbbbbb",-1);
//插入
r=mongoc_collection_insert(collection,0,insert,0,bserr);
if(!r){
console.log(r,bserr.code,bserr.err)
}
//更新
//从json文本创建udate的bson
json='{"time":1705890869470,"data":{"live_addr":"省县街道路2号1","live_street":"镇","name":"张三","phone_number":"1234567890","pid":"000000000000000000","temp_addr":"街道路2号1"},"code":200,"errMsg":null,"errCode":null,"msg":"success"}'
update=bson_new_from_json(json,-1,bserr);
if(!update){
console.log(update,"bson",bserr.code,bserr.err)
}
console.pause();
//用update的bson更新之前insert的bson
r=mongoc_collection_update (collection, 0, insert, update, 0, bserr)
if(!r){
console.log(r,bserr.code,bserr.err)
}
//销毁insert的bson
bson_destroy(insert);
//销毁update的bson
bson_destroy(update);
//查询
console.pause();
//创建query空bson
query = bcon_new();
console.pause();
json='{"data.name":"张三"}'
query=bson_new_from_json(json,-1,bserr)
console.pause()
console.more(1,true)
console.logPause(bson_as_json(query,0))
cursor = mongoc_collection_find(collection, 0, 0, 3, 0, query, 0, 0);
console.log("cursor", cursor);
//查询结果
var a=0;
result,a= mongoc_cursor_next(cursor, a);
console.log("result", result,a);
console.pause()
while (result) {
str=bson_as_json(a,0);
console.log(str);
result,a= mongoc_cursor_next(cursor, a);
}
//销毁query空bson
bson_destroy(query);
//删除
json='{"_id":"65af909e000000004f5f0803"}'
remove=bson_new_from_json(json,-1,bserr);
if(!remove){
console.log("转换json文本错误",bserr.code,bserr.err)
}
console.pause();
r=mongoc_collection_remove(collection,0,remove,0,bserr);
if(!r){
console.log(r,bserr.code,bserr.err)
}
//销毁remove的bson
bson_destroy(remove);
//销毁collection句柄
mongoc_collection_destroy(collection);
//销毁database句柄
mongoc_database_destroy(database);
//销毁client句柄
mongoc_client_destroy(client);
//销毁初始化
ab = mongoc_cleanup();
console.log(ab);
console.pause();
win.loopMessage();