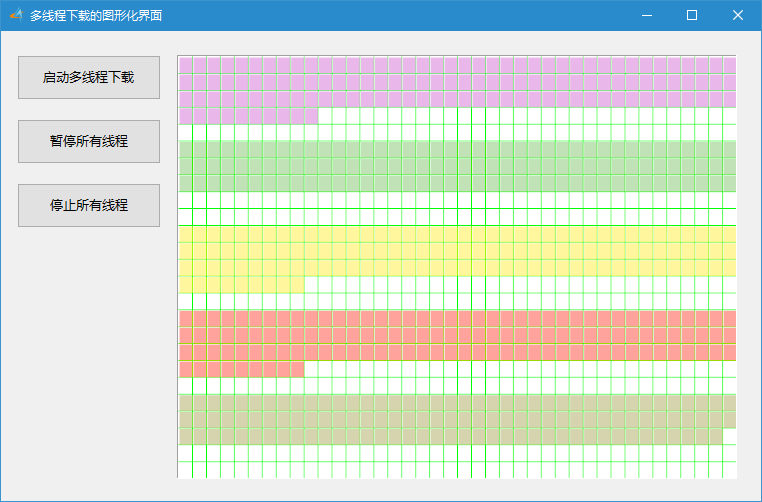
使用thread.manage来管理多线程,使用plus来绘制下载的可视化界面,用线程表在界面与线程池之间传递数据。
//模拟多线程下载的可视化界面
import win.ui;
/*DSG{{*/
var winform = win.form(text="多线程下载的图形化界面";right=759;bottom=469)
winform.add(
btnPause={cls="button";text="暂停所有线程";left=16;top=88;right=160;bottom=133;dl=1;dt=1;z=2};
btnStart={cls="button";text="启动多线程下载";left=16;top=24;right=160;bottom=69;dl=1;dt=1;z=1};
btnStop={cls="button";text="停止所有线程";left=16;top=152;right=160;bottom=197;dl=1;dt=1;z=3};
plus={cls="plus";left=176;top=24;right=736;bottom=448;clipBk=1;db=1;dl=1;dr=1;dt=1;edge=1;notify=1;repeat="center";z=4}
)
/*}}*/
import thread.manage; //导入线程管理器
manage = thread.manage(5) //指定线程池的最大线程数
//以下模拟一个文件的大小,及映射为图像的宽高信息
var totalSize = 1000;//下载文件的总大小
var rowNum = 40;
var colNum = totalSize / rowNum;
import thread.table; //导入线程表
var tt_DoneColor = thread.table(); //用来存储填充颜色
//初始化线程表
var ini_threadtables = function(){
for(i=1; totalSize; 1){
tt_DoneColor[i] = null;
}
}
//这是模拟下载的函数
var downloading = function(arr1,color,startNum,endNum,pieceSize){
if(!endNum) endNum = startNum + pieceSize -1; //自动截取分块大小
for(i=startNum; endNum; 1){
sleep(math.random(30,300)) //模拟网络下载花费的时间
arr1[i]= color; //修改线程表的数据
}
}
winform.btnStart.oncommand = function(id,event){
if(manage.busy()==true) return;
ini_threadtables(); iscancle = false;
//创建5个线程任务,模拟执行下载任务
var pieceSize = totalSize / 5 //设置每个线程下载的尺寸(将文件均分为5等分)
manage.create(downloading, tt_DoneColor, 0xEEE080E0/*淡紫色*/, 1,, pieceSize)
manage.create(downloading, tt_DoneColor, 0xEE65C200/*草绿色*/, pieceSize+1,, pieceSize)
manage.create(downloading, tt_DoneColor, 0xEEFFC212/*黄 色*/, pieceSize*2+1,, pieceSize)
manage.create(downloading, tt_DoneColor, 0xEEFF4100/*洋红色*/, pieceSize*3+1,, pieceSize)
manage.create(downloading, tt_DoneColor, 0xEEBDB76B/*卡其色*/, pieceSize*4+1,, pieceSize)
manage.waitClose() //等待所有线程结束
if(not iscancle) win.msgbox("下载结束","提示")
}
winform.btnPause.oncommand = function(id,event){
if(manage.busy()==false) return;
if(owner.text =="暂停所有线程"){
manage.suspend();
owner.text = "恢复所有线程"
}else {
manage.resume();
owner.text = "暂停所有线程"
}
}
winform.btnStop.oncommand = function(id,event){
if(manage.busy()==false) return;
var handles = manage.getHandles();
for(i=1;#handles;1){
thread.terminate(handles[i],0) //停止线程
}
iscancle = true;
win.msgbox("下载已取消","提示")
winform.btnPause.text = "暂停所有线程"
}
//画彩色小方块
winform.plus.onDrawContent = function(graphics,rc,txtColor,rcContent,foreColor){
graphics.clear(0xFFFFFFFF)
var w, h = rcContent.width(), rcContent.height();
var cell_w , cell_h = w / rowNum, h / colNum;
var brush = gdip.solidBrush()
for(i=1; totalSize; 1){
var x =(i-1) % rowNum;
var y = math.floor((i-1)/rowNum);
brush.color = tt_DoneColor[i] : 0x55CCCC00/*土黄色*/;
graphics.fillRectangle(brush, x*cell_w+1, y*cell_h+1, cell_w-1, cell_h-1);
}
return true;
}
//定时刷新plus
winform.plus.setInterval(
function(){
winform.plus.redraw();
},100
)
winform.show();
win.loopMessage();